Constructing Complex Queries with SQL Subqueries: Strategies and Examples
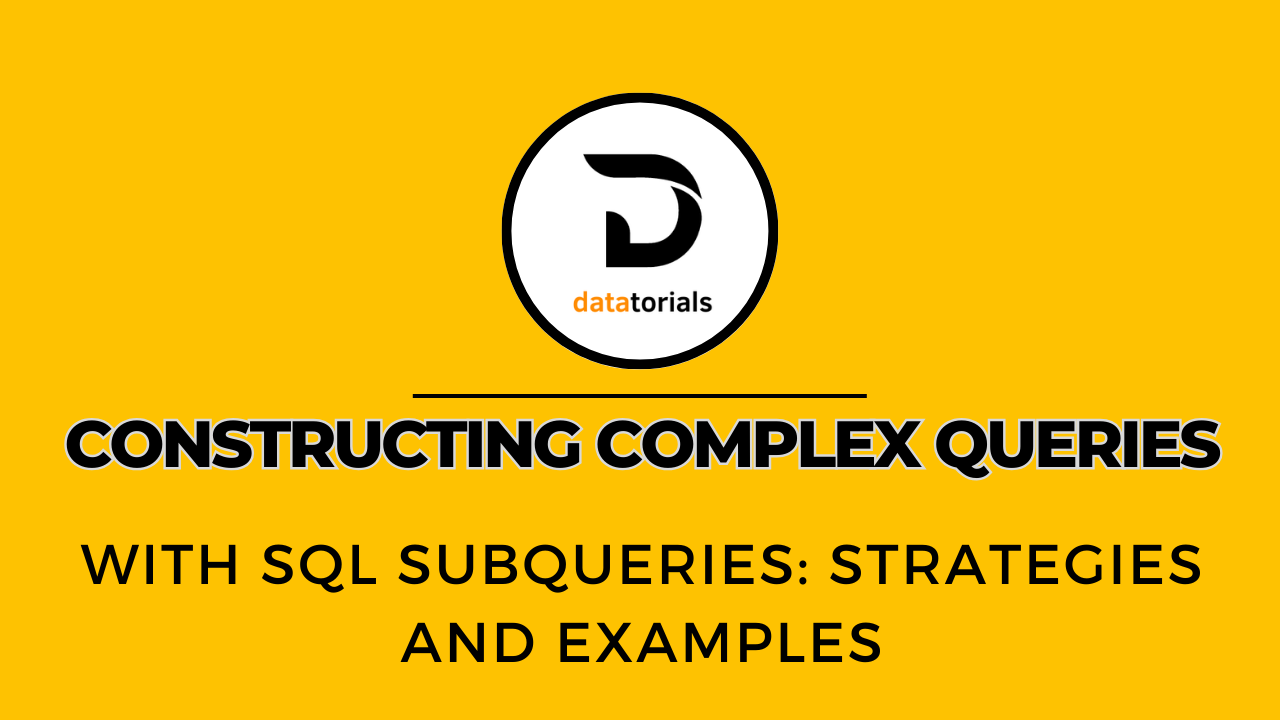
Introduction:
In the world of database querying, not all questions can be answered with simple SELECT statements. Enter SQL subqueries – a powerful tool for constructing complex queries that demand more intricate data manipulation.
In this blog post, we’ll delve into the art of utilizing SQL subqueries, explore various strategies, and provide real-world examples that demonstrate their versatility.
Understanding SQL Subqueries:
What Are Subqueries? A SQL subquery, also known as a nested query, is a query nested within another query. Subqueries allow you to fetch data based on the results of another query, making it possible to tackle complex scenarios.
Use Cases:
- Retrieving data from related tables
- Filtering data using aggregated results
- Performing calculations based on intermediate results
- Handling conditional logic
Strategies for Effective Subquery Usage:
- Use Subqueries as Data Sources: Embed subqueries in the FROM clause to treat their results as temporary tables. This approach simplifies complex queries and enhances readability.
- Leverage Subqueries for Filtering: Apply subqueries in WHERE and HAVING clauses to filter data based on specific conditions or aggregations from other tables.
- Utilize Correlated Subqueries: Correlated subqueries reference outer query columns, allowing dynamic interactions between the subquery and outer query.
- Optimize with EXISTS and NOT EXISTS: EXISTS and NOT EXISTS subqueries are efficient for checking the existence of related records. They return a boolean value indicating presence.
- Aggregate with Subqueries: Use subqueries with aggregate functions to retrieve aggregated results that feed into the outer query.
Real-World Examples:
- Fetching Product Details for High-Revenue Orders: Retrieve product details for orders with a total amount exceeding a certain threshold.
- Identifying Customers with No Orders: Find customers who haven’t placed any orders, using a subquery to compare customer IDs across tables.
- Calculating Employee Average Salaries per Department: Obtain average salaries for each department, leveraging subqueries to calculate totals and counts.
Example 1: Using Subqueries as Data Sources
SELECT products.product_name, order_details.unit_price
FROM products
JOIN (
SELECT order_id, product_id, unit_price
FROM order_details
) AS order_details
ON products.product_id = order_details.product_id;
Example 2: Leveraging Subqueries for Filtering
SELECT customer_id, order_date, total_amount
FROM orders
WHERE total_amount > (
SELECT AVG(total_amount)
FROM orders
);
Example 3: Utilizing Correlated Subqueries
SELECT employee_name
FROM employees e
WHERE EXISTS (
SELECT 1
FROM orders o
WHERE o.employee_id = e.employee_id
);
Example 4: Optimizing with EXISTS and NOT EXISTS
SELECT product_name
FROM products p
WHERE EXISTS (
SELECT 1
FROM order_details od
WHERE p.product_id = od.product_id
AND od.unit_price > 50
);
Example 5: Aggregate with Subqueries
SELECT department_id, department_name, average_salary
FROM departments d
JOIN (
SELECT department_id, AVG(salary) AS average_salary
FROM employees
GROUP BY department_id
) AS avg_salaries
ON d.department_id = avg_salaries.department_id;
Conclusion:
SQL subqueries are a powerful weapon in your query arsenal, empowering you to navigate complex data scenarios with finesse. By mastering the art of constructing queries with subqueries, you’ll open the door to advanced data retrieval, aggregation, and manipulation. Whether you’re dealing with intricate reporting needs or sophisticated data filtering, subqueries offer the versatility needed to extract meaningful insights from your database.